Imagine a world where everything works all the time, and there are no weird-ass errors to troubleshoot. Sounds boring, yeah? Good thing it’s not a real place.
Fantasies aside, sometimes things that have been working for ages just stop working. We ran into that case just recently with a project. This project has been live for many months, and suddenly started failing. (Note that the build passed, the deploy failed).
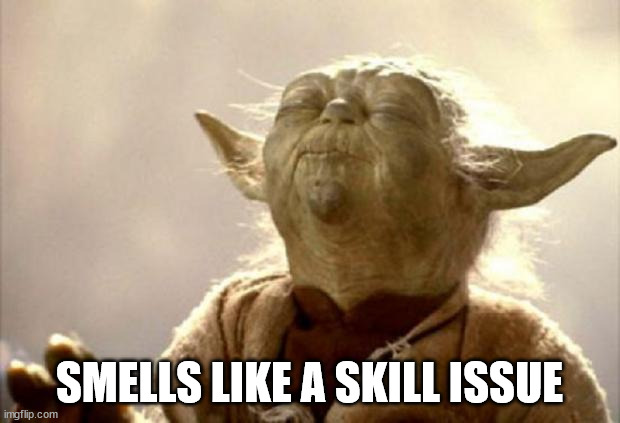
Here’s what Vercel was telling us:
An unexpected error happened when running this build. We have been notified of the problem. This may be a transient error. If the problem persists, please contact Vercel Support https://vercel.com/help
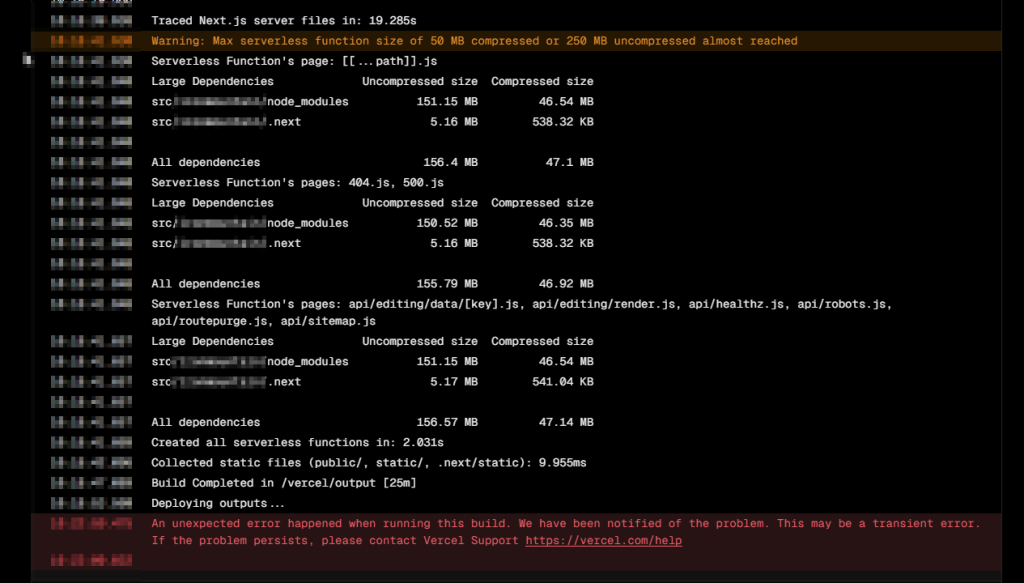
We waited. And then we opened a support ticket. Vercel’s response was not….ideal. They said “You should not do any SSG, or you should SSG the same number of items as when the build didn’t fail.
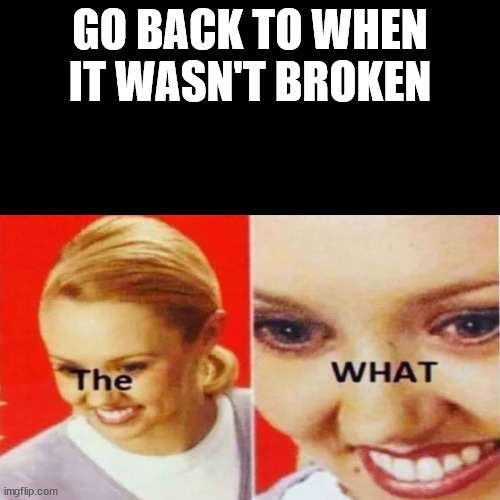
So, the “go back to the last good build” really wasn’t an option. That build, had, however, only SSG’d 6,000ish pages. We were wondering if these were somehow related, since that was the first thing Vercel Support went to. I did some ol’ fashioned googling and found this discussion on Vercel’s github: https://github.com/vercel/vercel/discussions/4009
The discussion basically says you get a deployment (not build) error when you have more than 8,000 SSG’d files in the deployment. If you check the links from that discussion, though, you see that the verbiage about limitations is gone from the official docs. Surely it’s not an issue anymore, right?
Not so much.
We modified the [[…path]].tsx to essentially limit the amount of paths returned from the sitemapFetcher. Something like this would work (See Line 76), but you’d want something more elegant to incorporate some business logic.
import { useEffect } from 'react';
import { GetStaticPaths, GetStaticProps } from 'next';
import NotFound from 'src/NotFound';
import Layout from 'src/Layout';
import {
RenderingType,
SitecoreContext,
ComponentPropsContext,
EditingComponentPlaceholder,
StaticPath,
} from '@sitecore-jss/sitecore-jss-nextjs';
import { handleEditorFastRefresh } from '@sitecore-jss/sitecore-jss-nextjs/utils';
import { SitecorePageProps } from 'lib/page-props';
import { sitecorePagePropsFactory } from 'lib/page-props-factory';
import { componentBuilder } from 'temp/componentBuilder';
import { sitemapFetcher } from 'lib/sitemap-fetcher';
const SitecorePage = ({
notFound,
componentProps,
layoutData,
headLinks,
}: SitecorePageProps): JSX.Element => {
useEffect(() => {
// Since Sitecore editors do not support Fast Refresh, need to refresh editor chromes after Fast Refresh finished
handleEditorFastRefresh();
}, []);
if (notFound || !layoutData.sitecore.route) {
// Shouldn't hit this (as long as 'notFound' is being returned below), but just to be safe
return <NotFound />;
}
const isEditing = layoutData.sitecore.context.pageEditing;
const isComponentRendering =
layoutData.sitecore.context.renderingType === RenderingType.Component;
return (
<ComponentPropsContext value={componentProps}>
<SitecoreContext
componentFactory={componentBuilder.getComponentFactory({ isEditing })}
layoutData={layoutData}
>
{/*
Sitecore Pages supports component rendering to avoid refreshing the entire page during component editing.
If you are using Experience Editor only, this logic can be removed, Layout can be left.
*/}
{isComponentRendering ? (
<EditingComponentPlaceholder rendering={layoutData.sitecore.route} />
) : (
<Layout layoutData={layoutData} headLinks={headLinks} />
)}
</SitecoreContext>
</ComponentPropsContext>
);
};
// This function gets called at build and export time to determine
// pages for SSG ("paths", as tokenized array).
export const getStaticPaths: GetStaticPaths = async (context) => {
// Fallback, along with revalidate in getStaticProps (below),
// enables Incremental Static Regeneration. This allows us to
// leave certain (or all) paths empty if desired and static pages
// will be generated on request (development mode in this example).
// Alternatively, the entire sitemap could be pre-rendered
// ahead of time (non-development mode in this example).
// See https://nextjs.org/docs/basic-features/data-fetching/incremental-static-regeneration
let paths: StaticPath[] = [];
let fallback: boolean | 'blocking' = 'blocking';
if (process.env.NODE_ENV !== 'development' && !process.env.DISABLE_SSG_FETCH) {
try {
// Note: Next.js runs export in production mode
// Cull list to 7,000 items, leaving room for misc items
paths = (await sitemapFetcher.fetch(context)).slice(0, 7000);
} catch (error) {
console.log('Error occurred while fetching static paths');
console.log(error);
}
fallback = process.env.EXPORT_MODE ? false : fallback;
}
return {
paths,
fallback,
};
};
// This function gets called at build time on server-side.
// It may be called again, on a serverless function, if
// revalidation (or fallback) is enabled and a new request comes in.
export const getStaticProps: GetStaticProps = async (context) => {
const props = await sitecorePagePropsFactory.create(context);
return {
props,
// Next.js will attempt to re-generate the page:
// - When a request comes in
// - At most once every 5 seconds
revalidate: 5, // In seconds
notFound: props.notFound, // Returns custom 404 page with a status code of 404 when true
};
};
export default SitecorePage;
Once we modifed this, and only generated about 7,000 files, our build magically went through. Our support case is ongoing and I’ll update this with results!
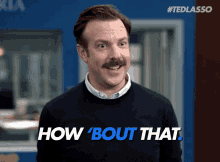

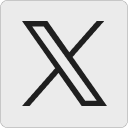


